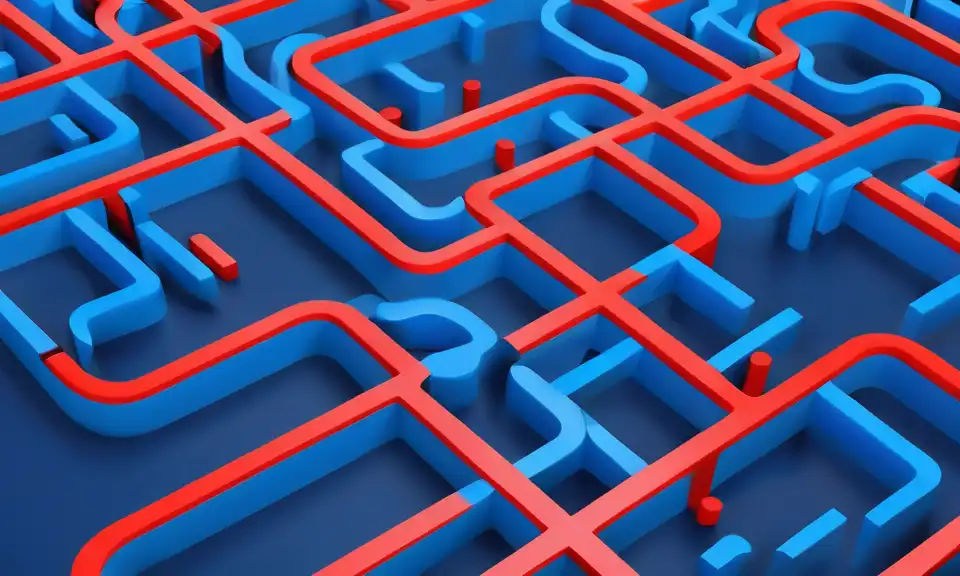
The Result Type: When errors can't be ignored.
When I started front end development, error handling was easy because I worked on a simple insurance website creating forms. The errors I cared about were relatively simple and could usually be sufficiently handled with a validation library or with a try catch block around network requests where I could set an error message in the catch block. Eventually, I changed jobs and got a chance to work on applications with more nuance in their error handling. When things go wrong, sometimes it matters beyond making your code stop to display a fatal error to the user. In many cases, we can salvage the user’s session by giving them a degraded experience that likely still meets their needs. In other scenarios, we may write utilities with errors that are intended to be used by many teams and we want other developers to handle the errors when they use our tools.
In this post, I first give an introduction to different kinds of errors you encounter when building software. After, I show how the Result Type is an excellent tool for handling errors on many projects.
A quick intro to errors
There are three kinds of errors that you generally need to worry about when building software:
- Checked Exceptions - When we believe errors are recoverable or are important to know about for developers using our function.
- Unchecked Exceptions - When we believe the error may happen but is unrecoverable, or at least generally not worth adding to our function signature.
- Invariant Violations - When we believe something should not be possible, or violates a fundamental assumption we’ve made about our project. When these errors occur, it’s good to pair them with a way of notifying your team through something like Sentry.
The terms “Checked” and “Unchecked” exceptions are especially common in many object oriented languages, so we’ll include a Java example.
Checked Exceptions
You’ll notice below that the method has throws JsonProcessingException as part of its method signature.
public void myMethod(Object object) throws JsonProcessingException {
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.writeValueAsString(object);
}
This is because JsonProcessingException is a checked exception which means that you must either handle the exception with try/catch or declare it in the method signature so that methods that call your method are also aware of the potential issue.
If you’re unsure of the utility of checked exceptions like the one above, just think about all the times that someone forgets to handle JSON.parse, or forgets that local and session storage operation in browsers can throw runtime exceptions if they are disabled by users. The purpose of checked exceptions is to get rid of surprise errors.
Unchecked Exceptions
If you’re a TypeScript developer, then you’re familar with the concept of unchecked exceptions — throwing errors in JavaScript works like an unchecked exception.
In unchecked exceptions, an error is thrown and no modifications are made to the type definition of the function the error lives in. In TypeScript, this is the default. In languages like Java, we have the choice to omit the exception from the method signature, and we likely choose to do so when errors happen that prevent a meaningful way forward in our program’s execution. Examples of this are when the client gives our server a payload that fails validation, or when a unique constraint is violated in our database. It’s easiest in these scenarios to throw a runtime exception and use tooling that maps them to appropriate status codes on our behalf.
Invariant Violations
Some may conflate invariant violations with unchecked exceptions, but I believe it’s a mistake to fail to see the differences between the two. The main difference is that invariant violations are:
- Expected to be impossible.
- Bad things can happen when the invariant doesn’t hold.
When invariant violations happen, we not only want to throw an exception that communicates that the error is unexpected, we also want to make our development teams aware of the error through alerting and error monitoring.
The Result Type: A Powerful Alternative
After examining the different types of errors we encounter, let’s explore a pattern that has gained popularity in many programming languages: the Result type. The benefit to the Result Type is that it provides a way to handle errors as data with utilities baked into its implementation that are standard among many languages that use Result.
What is the Result Type?
The Result type is a container that can hold either a successful value or an error. It’s a formal way to represent the outcome of an operation that might fail, without resorting to exceptions.
A simple implementation in TypeScript might look like this:
type Result<T, E> = {
success: true;
value: T;
} | {
success: false;
error: E;
};
This simple type allows us to return either a successful result with a value, or a failure result with an error. Let’s look at how we might use it:
function parseJSON<T>(json: string): Result<T, Error> {
try {
const parsed = JSON.parse(json);
return { success: true, value: parsed };
} catch (e) {
return { success: false, error: e as Error };
}
}
const result = parseJSON<User>(userJson);
if (result.success) {
console.log(result.value.name);
} else {
console.error(result.error.message);
}
Combining Checked and Unchecked Exception Handling
The Result type elegantly combines the benefits of both checked and unchecked exceptions:
-
Like checked exceptions, it makes errors explicit in the function’s return type. This forces developers to acknowledge the possibility of failure when they call your function.
-
Like unchecked exceptions, it doesn’t force callers to handle every possible error immediately. They can choose to pass the Result along to be handled at a more appropriate level.
Let’s see a more complex example that demonstrates these benefits:
// A library function that returns a Result
function fetchUserData(userId: string): Result<UserData, ApiError> {
// Implementation details...
}
// Application code using the library function
function getUserProfile(userId: string): Result<UserProfile, AppError> {
const userResult = fetchUserData(userId);
if (!userResult.success) {
// Map the library error to an application-specific error
return {
success: false,
error: new AppError(`Failed to fetch user: ${userResult.error.message}`)
};
}
// Process the successful result
const userData = userResult.value;
return {
success: true,
value: {
name: userData.name,
email: userData.email,
}
};
}
Leaving Room for Invariant Violations
While the Result type is excellent for handling expected errors, it’s important to note that it doesn’t replace the need for handling invariant violations. Invariants, by definition, should never be violated, and when they are, it represents a fundamental bug in our system.
For invariant violations, we should still use traditional exception throwing along with monitoring:
function processVerifiedUser(user: VerifiedUser) {
if (!user.isVerified) {
// This should be impossible since we're accepting a VerifiedUser
const error = new InvariantViolationError("Unverified user in verified context");
captureException(error); // Send to monitoring service like Sentry
throw error;
}
// Normal processing continues...
}
Benefits of Using the Result Type
-
Explicit error handling: Errors become part of your function signatures, making them impossible to ignore accidentally.
-
Type safety: You get compile-time guarantees that errors are handled appropriately.
-
No exception stack unwinding: Control flow remains predictable and easier to reason about.
-
Composability: Results can be easily composed, chained, and transformed in functional programming patterns.
-
Better testing: Functions that return Results are typically easier to test than those that throw exceptions.
Libraries and Frameworks
Many languages have mature libraries implementing the Result pattern:
- Rust has
Result<T, E>
built into its standard library - TypeScript has libraries like
neverthrow
andts-results
- JavaScript has libraries like
true-myth
andresult-js
- Kotlin has
Result
in its standard library - Swift has
Result<Success, Failure>
Conclusion
The Result type offers a powerful alternative to traditional exception handling by making errors explicit and recoverable while preserving normal control flow. It combines the best aspects of both checked and unchecked exceptions:
- Like checked exceptions, errors are explicit and can’t be silently ignored
- Like unchecked exceptions, error handling can be deferred to an appropriate level of your application
Meanwhile, for true invariant violations that represent bugs in your system, traditional exceptions paired with monitoring remain the appropriate solution.
By adopting this pattern, your error handling becomes more explicit, your function signatures become more honest about potential failures, and your code becomes more reliable and maintainable.