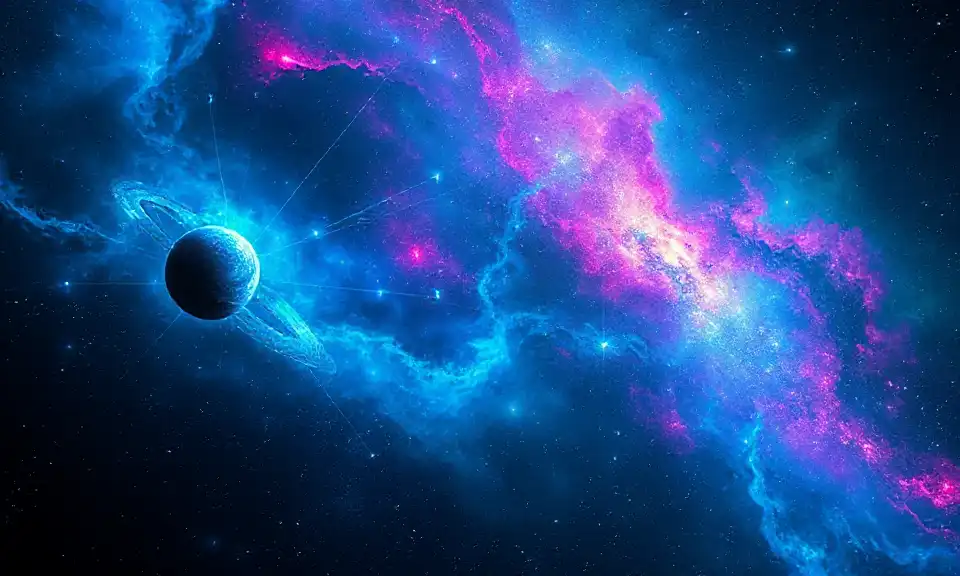
Useful type predicates: assume only what you can prove
Type predicates are an advanced feature in TypeScript - you won’t learn them on day 1. When you finally learn them, you might know something that many on your team do not yet understand. It can be tempting to conflate advanced techniques with techniques that have a positive impact on your code. If you stop to think about type predicates, you’ll find that they are easily abused. Type predicates allow you to give arbitrary evidence to prove that a value is a certain type.
The example below is contrived to get the point across. Just because a value is an object that happens to have a name property doesn’t mean it’s a User.
function isUser(value: unknown): value is User {
return typeof value === 'object' && value !== null && 'name' in value
}
We might be temped to make the chances of a conflict less likely by narrowing the allowed inputs to a set of types. In the example below, value is narrowed to User or Bot. In our app today, no Bot has a property called “name.”
function isUser(value: User | Bot): value is User {
return 'name' in value
}
The problem is obvious — it’s totally possible that a Bot might someday include a name property and it’s on our engineering team to just know that they have to update this predicate in our system. Such a distinction between a bot and a user is likely better represented by a discriminated union, not a type predicate.
To be fair, a type predicate of this kind isn’t always wrong. We may have two or more sets of objects that are differentiated by a fundamental truth of our product that’s difficult to distinguish with a discriminant alone, but this is extremely rare.
Time for the punchline. It actually can be useful to have a type predicate like the one above, but only if the predicate proves the outcome.
The example from before only supplied proof that a User or a Bot would have a name. The conclusion that, if value has a name property, then it is necessarily a User is not necessarily true even if it happens to be true today. A better type predicate would tell you whether or not name is included in the value provided regardless of whether its a Bot or a User. Here’s what that could look like.
function hasName<T extends { name: string }, U extends User | Bot>(value: U): value is T & U {
return 'name' in value;
}
Now we’ve made a guarantee based only on what we’ve proven to be true so that if a Bot someday has a name, it will pass the predicate.
I asked AI to provide a non-trivial example of what’s been described. You’ll notice that the type predicate demonstrates editability based on discriminants that describe editable document types.
// These are our base interfaces
interface BaseDocument {
id: string;
createdAt: Date;
updatedAt: Date;
}
interface DraftDocument extends BaseDocument {
status: 'draft';
content: string;
lastEditor: string;
}
interface PublishedDocument extends BaseDocument {
status: 'published';
content: string;
publishedAt: Date;
version: number;
}
interface ArchivedDocument extends BaseDocument {
status: 'archived';
archivedAt: Date;
archivedBy: string;
reason: string;
}
// Union type representing all document states
type Document = DraftDocument | PublishedDocument | ArchivedDocument;
// Type predicate that actually proves what it claims
function isModifiableDocument<T extends Document>(doc: T): doc is T & (DraftDocument | PublishedDocument) {
// We're checking the fundamental property that determines modifiability
// This is a truth about our domain model, not an arbitrary property check
return doc.status === 'draft' || doc.status === 'published';
}
// Usage example
function updateDocumentContent(doc: Document, newContent: string): Document {
if (isModifiableDocument(doc)) {
// TypeScript now knows this is either DraftDocument or PublishedDocument
// Both of which have 'content' property
return {
...doc,
content: newContent,
updatedAt: new Date()
};
} else {
// TypeScript knows this is an ArchivedDocument
throw new Error(`Cannot modify archived document ${doc.id}`);
}
}