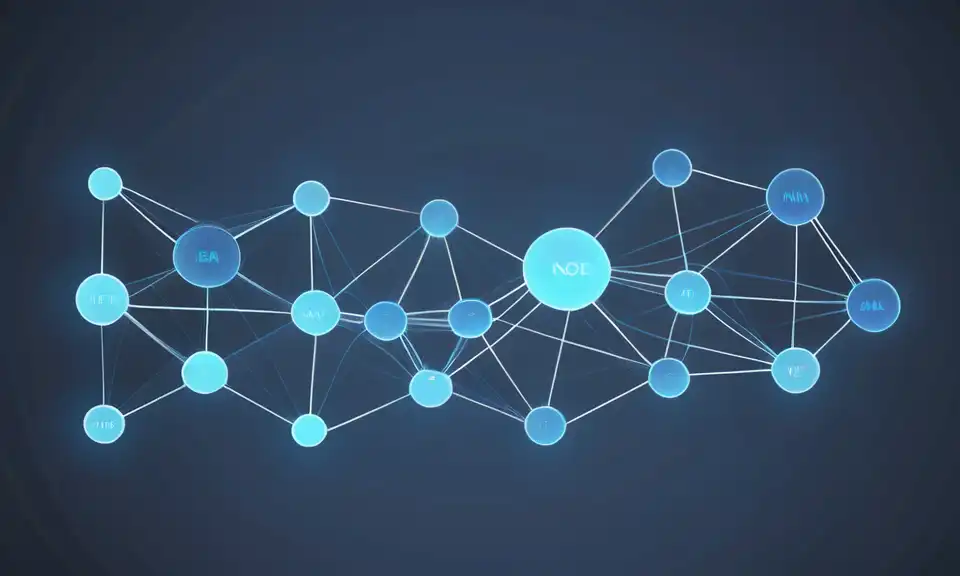
Reflections on complex Front End Events and State
It’s been right around a year since I’ve started obsessing over events and state management — I’ve created several posts on events and state, and each one reflects progress I’ve made along the way in my thinking, but I’ve never taken the time to express any one post to the best of my ability. Each one has been a snapshot into my thoughts at that time, but none of them can be described as persuasive.
Starting with Elm
To give some context, learning Elm was the single most important factor in growing my thinking in terms of event/state management (among other things). While Elm isn’t exactly widely used, its impact on the front end world is greater than people realize.
Redux was inspired by Elm and the Redux site even recommends tinkering with Elm to understand how Elm’s constraints offer stability. Elm advertises no runtime errors in practice which is mind blowing coming from the world of JavaScript where runtime errors happen frequently. Between Elm’s purely functional approach, its type system, and the Elm Architecture (which is its equivalent to Redux), developers are almost always safe from accidentally creating runtime errors.
As a JavaScript developer, I wanted the benefits Elm offered in my projects at work — some of which had become fragile. Good things happened when I started applying what I learned from Elm:
- The bugs decreased drastically when I started leaning into concepts from functional programming such as immutability and pure functions.
- I adhered to TypeScript as strictly as possible and used stricter settings to protect my team from type related runtime errors. I also started to lean into type variants and exhaustive matching which are things I’d learned from Elm.
- I began imitating Redux and the Elm Architecture to handle events and state updates.
The benefits from 1 and 2 are easier to understand and explain than 3. I believe that Redux and the Elm Architecture provide stability to front end applications. The feeling of playing JavaScript Jenga where changing one small item might cause some unforeseen thing to break has largely gone away even on the most complicated work I’m involved with. At the same time, I’ve had a hard describing how and why to other developers.
Unpacking the “Why” of Redux
This is my best and most thought out explanation of why Redux works. For starters, it’s almost impossible to sandbox the benefits of Redux because small apps that we use for sandboxing examples don’t need Redux. Redux shines in the midst of chaotic product requirements involving interdependent and deeply nested components. It is a tool for managing complexity. In other words, if you try to show the benefits of Redux with a counter app, it’ll seem like a ridiculous solution to a simple problem.
Structure
So what is it that complex apps need that something like a counter app don’t? For starters it’s structure.
Speaking of structure, I’ve done a bit of back end work and back end engineers will generally follow a pattern that adheres to something like this in its most simple form: 1) Our controllers create end points to expose. These controllers serialize and deserialize inbound and outbound data as well as validate requests. 2) Service classes then receive data from the controllers to do business logic. 3) Data Access Objects are then created to interact with data stores.
Why bother with these different pieces? Why not just throw everything into the same file or break our files into smaller pieces when we feel one is getting too large without the strict categories? The answer is that these different kinds of classes provide structure, and structure makes it easier to think about complicated work.
So how does Redux provide structure? Let’s start by imagining an app without Redux. We start with something like a button that triggers some functionality, so we write a function and put it into the body of our component. Then another element has to do something else, so we add a function and put it in the body of our component. Each of these either updates state and/or triggers side effects. As our component grows in size, we break it apart into smaller components to try to give ourselves something easier to think about, but the smaller pieces are still interconnected even if they live in smaller files. The smaller files offer some benefits such as giving us something easier to test, but the interconnected, and sometimes reactive, dependencies are still something to deal with. All in all, we could say that there’s likely some structure in this design, but we haven’t created limitations, we haven’t defined how data is allowed to flow, and have logic spread through components in no particular order for events that exist somewhere in the call stack.
With Redux, we know exactly where to expect side effects, logic for events, and interpretation of state to live. Redux provides a well defined path for events to resolve by providing an enumerated list of actions for all events, a side effect manager to resolve side effects, a reducer to handle event logic especially as it pertains to impact on state, and selectors to synthesize and interpret state’s impact on subscribing components. In this way, predictability is born out of structure — Coding no longer has to be a game of jenga (or at least not quite like it was).
Unidirectional thinking
A second benefit Redux provides is unidirectional thinking. For our purposes, this means there’s a clear distinction between the code that updates state and the code which consumes state. Without something like Redux, there’s no pattern that provides a distinction between interpreting state and updating state. We commonly have to go back and forth in our call stack to understand the impacts of some series of state updates to get to the cause of a problem. We can look at a component’s functions without immediately knowing if they handle events, perform some side effect, or interpret state — In other words, there’s bidirectional thinking at best and no clear direction in which to think at worst.
With Redux, we clearly define impacts of events as actions which are dispatched. Each action is enumerated so there’s no surprises. Actions can have side effects to resolve or they can go straight to a reducer. Selectors are at the end waiting to create fresh computations based on relevant state changes. If this sounds similar to the structured benefits from before, it’s because it’s almost the same. The structure provides a unidirectional way to think about events and state rather than working in the chaos of throwing all our functions together regardless of what they do.
Thinking in Events
Another major benefit to Redux is that it invites us to think in terms of events which serve as inputs from the outside world. The opposite of this is thinking in terms of state getters and setters. The model for thinking of getters and setters is more concerned with making existing state align with outcomes regardless of how the state model is defined. On the other hand, thinking in events means
- We design our state model to reflect the direct outcomes of events. We no longer necessarily seek to define state values in terms of their impact on subscribers — that’s the job of selectors. The state values represent the intended impacts of events. If these seem like the same thing, consider this example. When a user clicks a login button, their intended impact is to authenticate. The outcomes on the rest of the app can be computed from selectors which may decide whether or not to display a spinner, or whether or not to disable buttons on the page until authentication is done.
- The organization of the code now explicitly gives space for each event to be handled. Using an action in more than one place in our code should be done sparingly because each action should represent one event. The logic behind each of these actions is allowed to be shared, but the shared space is not the same as the space dedicated for the event itself. The benefit to this is that we can more safely add logic to the constituent side effect manager, reducer, and selectors represented by the action without having to worry about unintended consequences. This is case where reusability makes it harder to think about.
Breaking free of Object Oriented State Management
In OOP, each object is allowed to own its own state. These objects can be come dependent on the state of other objects. You may not think this is a problem in your React project with its “functional” components (which is actually called a function component by the React Docs), but it’s the exact model we adopt when our goal is to create many small components each with their own small amount of state.
Here is a link to a timestamp from a popular OOP is bad talk. Give it a watch and you’ll see how the problem OOP has had with state management mirrors our problems in React. The only time the problems described here are not an issue is when dealing with simple state and events that can fit into just a few components or are not interconnected: https://youtu.be/QM1iUe6IofM?t=1275
All in all, I’ve thought a lot about the proper application of Redux or patterns like Redux to achieve stability in front end applications. The concepts mentioned above may not apply to many front end projects you work on if what you work on is simple. The ability to create simple apps with idiomatic JavaScript/TypeScript isn’t enough to be an excellent front end engineer. We should be inspired by hard problems and do our best to learn to solve them well.